Travel management system - Entity-relationship diagram example
This ER diagram illustrates a Travel Management System involving Customers, Bookings, Destinations, Hotels, and Flights. Customers make bookings, each associated with a destination. Destinations have hotels and flights linked to them. The relationships between these entities demonstrate how customers make bookings for destinations, and how destinations are connected to hotels and flights, facilitating travel arrangements.
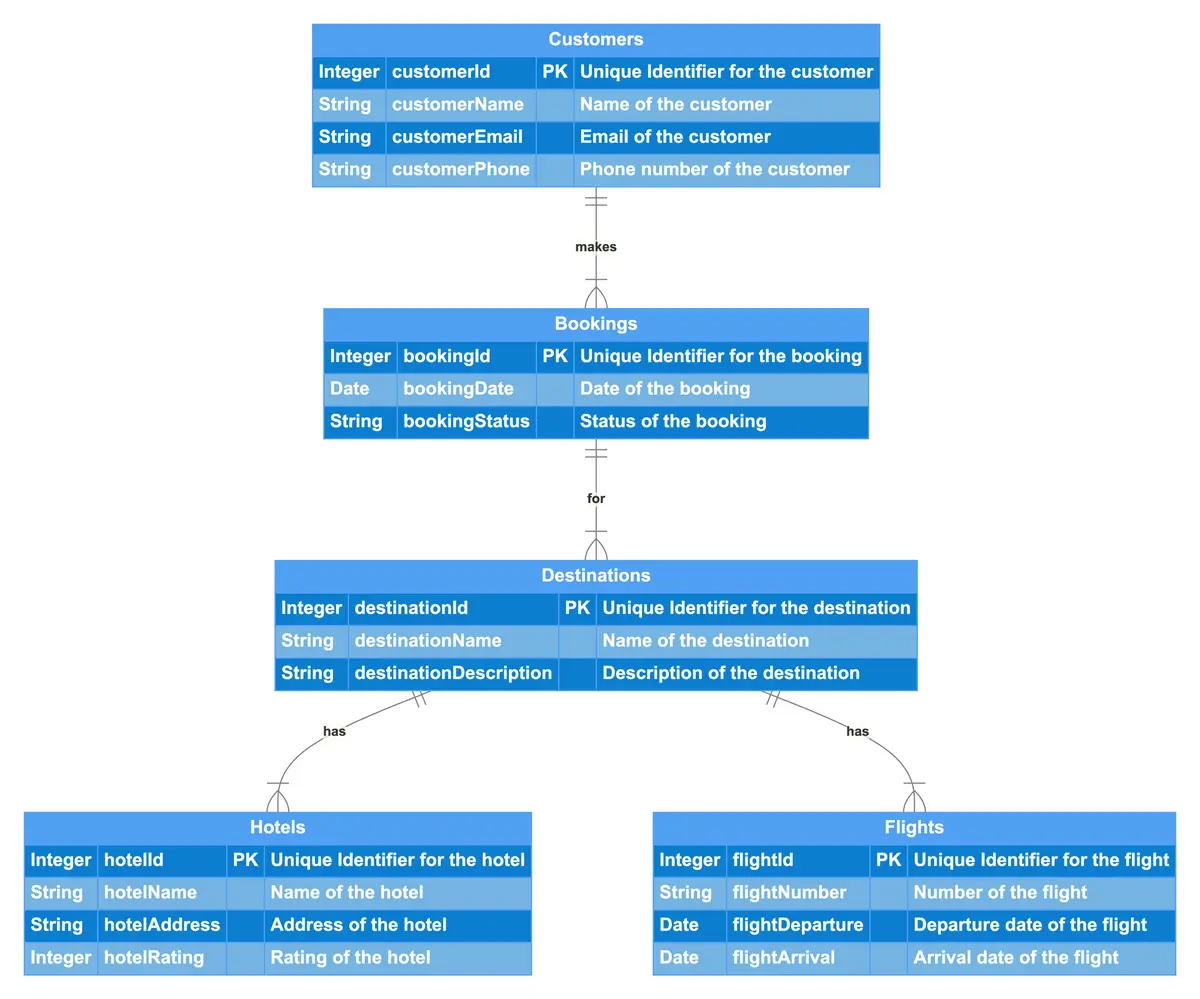

Edit this diagram in Gleek
Travel management system diagram code in gleek.
Integer customerId PK "Unique Identifier for the customer"
String customerName "Name of the customer"
String customerEmail "Email of the customer"
String customerPhone "Phone number of the customer"
Integer bookingId PK "Unique Identifier for the booking"
Date bookingDate "Date of the booking"
String bookingStatus "Status of the booking"
Destinations
Integer destinationId PK "Unique Identifier for the destination"
String destinationName "Name of the destination"
String destinationDescription "Description of the destination"
Integer hotelId PK "Unique Identifier for the hotel"
String hotelName "Name of the hotel"
String hotelAddress "Address of the hotel"
Integer hotelRating "Rating of the hotel"
Integer flightId PK "Unique Identifier for the flight"
String flightNumber "Number of the flight"
Date flightDeparture "Departure date of the flight"
Date flightArrival "Arrival date of the flight"
Customers {1}-makes-{1..n} Bookings
Bookings {1}-for-{1..n} Destinations
Destinations {1}-has-{1..n} Hotels
Destinations {1}-has-{1..n} Flights
About ER diagrams
We often make an entity-relationship (ER) diagram, ERD, or entity-relationship model, in the early stages of designing a database. An ERD is perfect for quickly sketching out the elements needed in the system. The ERD explains how the elements interact. ER diagrams can be shared with colleagues. Their simplicity makes them ideal even for non-technical stakeholders.
Similar ER diagram examples
Online store entity-relationship diagram
Simple ER diagram example with Chen notation
Employee management system entity-relationship diagram
E-commerce website ER diagram
Inventory management system ER diagram
Library management system ER diagram
Banking system entity-relationship diagram
College management system ER diagram
Online shopping entity-relationship diagram
Student management system entity-relationship diagram
Library management system ER diagram with Chen notation
Hospital management system entity-relationship diagram
Simple order process entity-relationship diagram

Complete Online Tourism Management System in PHP MySQL Free Source Code

Table of Contents
Project: Complete Online Tourism Management System in PHP MySQL Free Source Code
Welcome to our comprehensive guide on building an Online Tourism Management System in PHP and MySQL ! In this project, we will show you how to create a robust system that handles everything from user registration to managing tours, inquiries, and bookings.
What is an Online Tourism Management System?
An Online Tourism Management System is a web-based application that allows tourism businesses to manage their operations online. It typically includes features for user registration, managing inquiries, bookings, tours, and user management. By centralizing these functions, businesses can streamline their operations and provide a seamless experience to their customers.
Objective of an Online Tourism Management System
The primary objective of an Online Tourism Management System is to simplify and automate the various tasks involved in managing a tourism business. This includes managing bookings, handling inquiries, and providing a platform for customers to explore and book tours online. By providing these tools, businesses can improve efficiency, reduce manual work, and enhance the overall customer experience.
Description of Tourism Management System
A Tourism Management System is a comprehensive solution that covers all aspects of managing a tourism business. It includes features for managing tours, handling inquiries, processing bookings, and managing customer information. Additionally, it may include features for generating reports, analyzing data, and integrating with other systems such as payment gateways and accounting software.
How to Build a Tourism Management System in PHP MySQL
To build a Tourism Management System in PHP MySQL, you can follow these steps:
- Set Up Your Development Environment: Install a web server (e.g., Apache), PHP, and MySQL on your local machine or a server.
- Design Your Database: Create a database schema to store information such as tours, bookings, customers, and inquiries.
- Develop Your Application: Write PHP code to create the functionality for managing tours, bookings, inquiries, and user registration.
- Implement Security Measures: Implement security measures such as input validation, SQL injection prevention, and user authentication to protect your system from vulnerabilities.
- Test Your Application: Test your application thoroughly to ensure that it functions correctly and meets the requirements of your tourism business.
- Deploy Your Application: Once you have tested your application, deploy it to a production environment where it can be accessed by your customers.
How to Create a Travel Management System Project
Creating a Travel Management System project involves the following steps:
- Define Your Requirements: Identify the features and functionality you need in your Travel Management System, such as user registration, booking management, and tour management.
- Design Your System: Create a design for your system, including the user interface, database schema, and system architecture.
- Develop Your System: Write the code for your system, implementing the features and functionality you identified in the requirements phase.
- Test Your System: Test your system to ensure that it functions correctly and meets the needs of your business and users.
- Deploy Your System: Deploy your system to a production environment where it can be used by your customers.
Features of the Tourism Management System Project
Our Online Tourism Management System in PHP MySQL offers a wide range of features to make your tourism business thrive:
1. Dashboard
- Provides a quick overview of key metrics and activities.
2. User Registration
- Allows users to create accounts and manage their profiles.
3. Manage Inquiries
- Helps in handling and responding to inquiries from potential customers.
4. Manage Booking
- Enables efficient management of bookings, including approvals and cancellations.
5. Manage Tours
- Allows the addition, modification, and deletion of tour packages.
6. User Management
- Administer users and their access levels.
7. Manage Issues
- Handle and resolve customer issues effectively.
8. Manage Pages
- Easily edit and manage website content pages.
9. Approve/Cancel Booking
- Streamline the booking approval process.
10. Pending Booking
- Keep track of pending bookings for quick action.
11. 100% Responsive
- Ensure a seamless experience across all devices.
Screenshot of the Home Page

online tourism management system in php
Online Tourism Management System Flowchart
The flowchart represents the flow of interactions within an Online Tourism Management System in PHP MySQL (OTMS), detailing the actions and decisions users and administrators can take within the system. Here’s a description of the flowchart:
- Users can log in or register to access the system.
- Depending on their user type (customer or admin), they will be directed to different sections of the system.
- Customers can view available tours and make inquiries about them.
- They can also make bookings for tours and manage their bookings, such as viewing booking details and receiving booking confirmations.
- Admins have access to a dashboard where they can manage various aspects of the system.
- They can manage users, including adding, editing, and deleting user accounts.
- Admins can also manage tours, such as adding new tours, editing existing tours, and deleting tours.
- They can manage bookings, including viewing booking details and approving or canceling bookings.
- Admins can also manage inquiries, viewing inquiries and responding to them.
- Additionally, admins can manage issues, viewing and resolving any issues that arise.
- Finally, admins can manage website pages, including viewing and editing content pages.
- The flowchart illustrates the flow of information within the system, showing how users and admins interact with different components to perform various action

This flowchart provides a detailed overview of the functionalities of the Online Tourism Management System, highlighting the interactions between users and administrators and the processes involved in managing tours, bookings, inquiries, and other aspects of the system.
Online Tourism Management System ER Diagram
The Entity-Relationship (ER) Diagram for the Online Tourism Management System (OTMS) represents the entities and their relationships within the system. Here’s a detailed description of the diagram:
- The User entity represents users of the system, including their userID, username, password, email, and userType (which is a foreign key referencing the UserType entity).
- The UserType entity defines the type of user, such as customer or admin.
- The Booking entity represents bookings made by users, including bookingID, tourID (foreign key referencing Tour entity), userID (foreign key referencing User entity), bookingDate, and status.
- The Tour entity represents tours available in the system, including tourID, tourName, description, location, and price.
- The Inquiry entity represents inquiries made by users, including inquiryID, userID (foreign key referencing User entity), inquiryMessage, inquiryDate, responseMessage, and responseDate.
- The Issue entity represents issues reported by users, including issueID, userID (foreign key referencing User entity), issueMessage, issueDate, and status.
- The Page entity represents website pages, including pageID, pageTitle, and content.
- The Admin entity represents administrators of the system, including adminID, username, and password. Admins can manage pages.

This ER Diagram provides a comprehensive view of the data structure and relationships within the OTMS, detailing how users interact with the system, make bookings, inquiries, and reports, and how administrators manage pages.
To run this project you must have installed a virtual server i.e XAMPP on your PC. Online Tourism Management System in PHP with source code is free to download, Use for educational purposes only!
After Starting Apache and MySQL in XAMPP, follow the following steps
1st Step : Extract file 2nd Step: Copy the main project folder 3rd Step: Paste in xampp/htdocs/
Now Connecting Database
4th Step: Open a browser and go to URL “http://localhost/phpmyadmin/” 5th Step: Then, click on the databases tab 6th Step: Create a database naming “tms” and then click on the import tab 7th Step: Click on browse file and select “tns. sql ” file which is inside the “db” folder 8th Step: Click on go.
After Creating Database ,
9th Step: Open a browser and go to URL “http://localhost/onlinetourism”
Project Demonstration
Have a look at the video below for the project demonstration.
Related Projects: Online Class Scheduling System in PHP MySQL , Complete Class Scheduling System (Timetable generator) using Genetic Algorithms in C# and MS SQL , Complete College School Management System using Python Django , Complete School Management System using PHP MySQL , Inilabs School Express : Complete School Management System Software , Advanced School Management System with Complete Features , School File Management System using PHP/MySQLi , Online School Attendance Management System in PHP MySQL, Complete Web-Based School Management System using PHP MySQL , Complete School ERP System , Complete Multi Branch School Management System using PHP MySQL
Free Download Complete Online Tourism Management System in PHP MySQL Source Code
- Related Articles

Complete Teacher Submission and Monitoring System in PHP MySQL Free Download

Complete Car Wash Booking System in PHP MySQL with Mobile App Free Download

Student Timetable Management System in PHP MySQL Free Download
![Complete Pay Per Click Platform Script in PHP MySQL Free Download [Updated] 11 complete pay per click platform script](https://www.campcodes.com/wp-content/uploads/2021/06/complete-pay-per-click-platform-script-300x186.jpg.webp)
Complete Pay Per Click Platform Script in PHP MySQL Free Download [Updated]

This is a free education portal. You can use every source code in your project without asking permission to the author. Share our website to everyone to make our community of programmers grow more.
please tell me the password and user name of admin pannel

Hello bhai updata wala ka prpper run nahi ho raha hai please help me or make a vid..

Please translate in English.

Brother I am getting an error as “Connection failed: Access denied for user ‘root’@’localhost’ (using password: YES)” and once I put “GRANT ALL PRIVILEGES ON tms.* TO ‘root’@’localhost’ IDENTIFIED BY ‘Ansari@123’; FLUSH PRIVILEGES;” this in my configuration file it shows me a blank screen on the browser

hello brother I am getting an error as “Connection failed: Access denied for user ‘root’@’localhost’ (using password: YES)” but when I put “GRANT ALL PRIVILEGES ON tms.* TO ‘root’@’localhost’ IDENTIFIED BY ‘Ansari@123’; FLUSH PRIVILEGES;” in my config file I am getting a blank screen on the browser.

Please explain project for interview purpose.

What do you mean?
can you provide project report?

user and password for config
where is the admin details please?

thank you this is amezing website !! i am very happy!! again thank you!

how to change the background image
how can i change the background image pleas tell me it’s urgent
guys what you have done is very use full and appriatiate think things

im also admin login problem plz help

This project is very useful for education purpose. My heart full thanks to the demo video really amazing….But now the video is not visible…

i cant login to adminlogin the user and the password not correct need your help

Please check your admin localhost url.

how do i check?
same problem for me
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Activity Diagram (UML)
- Amazon Web Services
- Android Mockups
- Block Diagram
- Business Process Management
- Chemical Chart
- Cisco Network Diagram
- Class Diagram (UML)
- Collaboration Diagram (UML)
- Compare & Contrast Diagram
- Component Diagram (UML)
- Concept Diagram
- Cycle Diagram
- Data Flow Diagram
- Data Flow Diagrams (YC)
- Database Diagram
- Deployment Diagram (UML)
- Entity Relationship Diagram
- Family Tree
- Fishbone / Ishikawa Diagram
- Gantt Chart
- Infographics
- iOS Mockups
- Network Diagram
- Object Diagram (UML)
- Object Process Model
- Organizational Chart
- Sequence Diagram (UML)
- Spider Diagram
- State Chart Diagram (UML)
- Story Board
- SWOT Diagram
- TQM - Total Quality Management
- Use Case Diagram (UML)
- Value Stream Mapping
- Venn Diagram
- Web Mockups
- Work Breakdown Structure
New ER DIAGRAM FOR ONLINE TRAVEL AGENCY [classic]

You can easily edit this template using Creately. You can export it in multiple formats like JPEG, PNG and SVG and easily add it to Word documents, Powerpoint (PPT) presentations, Excel or any other documents. You can export it as a PDF for high-quality printouts.
- Flowchart Templates
- Org Chart Templates
- Concept Map Templates
- Mind Mapping Templates
- WBS Templates
- Family Tree Templates
- VSM Templates
- Data Flow Diagram Templates
- Network Diagram Templates
- SWOT Analysis Templates
- Genogram Templates
- Activity Diagram Templates
- Amazon Web Services Templates
- Android Mockups Templates
- Block Diagram Templates
- Business Process Management Templates
- Chemical Chart Templates
- Cisco Network Diagram Templates
- Class Diagram Templates
- Collaboration Diagram Templates
- Compare & Contrast Diagram Templates
- Component Diagram Templates
- Concept Diagram Templates
- Cycle Diagram Templates
- Data Flow Diagrams(YC) Templates
- Database Diagram Templates
- Deployment Diagram Templates
- Entity Relationship Diagram Templates
- Fishbone Diagram Templates
- Gantt Chart Templates
- Infographic Templates
- iOS Mockup Templates
- KWL Chart Templates
- Logic Gate Templates
- Mind Map Templates
- Object Diagram Templates
- Object Process Model Templates
- Organizational Chart Templates
- Other Templates
- PERT Chart Templates
- Sequence Diagram Templates
- Site Map Templates
- Spider Diagram Templates
- State Chart Diagram Templates
- Story Board Templates
- SWOT Diagram Templates
- T Chart Templates
- TQM - Total Quality Management Templates
- UI Mockup Templates
- Use Case Diagram Templates
- Value Stream Mapping Templates
- Venn Diagram Templates
- Web Mockup Templates
- Y Chart Templates
Related Templates
- SQL Cheat Sheet
- SQL Interview Questions
- MySQL Interview Questions
- PL/SQL Interview Questions
- Learn SQL and Database
How to Design ER Diagrams for Booking and Reservation Systems
Booking and reservation systems act as information on the systems that are software-based for the process of reserving services , accommodation , or resources . From hotels and flights to restaurants and diners, interactive online booking systems simplify the relationship between customers and service providers.
A very important step in the process of designing a system of this kind is ER diagram creation. This diagram will outline the database structure. This article helps you to explore ER diagrams designed for booking and reservation systems by considering things like entities/relationships/attributes necessary for the system’s smooth running.
Database Design for Booking and Reservation Systems
This platform includes a booking and reservation system that supports all aspects of handling bookings , and payments , as well as user management and interactions. Users are able to register and browse through the list of services. The system does all in all servicing related listings, as such, users are able to perform their preferences based on reviews .
This system, with functionalities like invoice generation, payment record monitoring, and personalized notice features, creates a convenient ambiance for the reservation user and manager.
Booking and Reservation Systems Features
User Management
- Secure registration, login, and profile management.
- Access to booking history for users.
Service Management
- Comprehensive service listings with search and filtering options.
- Detailed service descriptions and pricing.
Booking Management
- Easy booking creation, modification, and cancellation.
- Real-time status updates and notifications for bookings.
Review and Rating System
- User reviews and ratings for services.
- Moderation tools for review quality control.
Location Management
- Listing of service locations with search functionality.
Payment Processing
- Secure payment gateway integration with multiple payment options.
- Payment history tracking for users.
Cancellation and Refund Management
- User-friendly process for cancellations and refunds.
- Efficient handling and communication of cancellation/refund status.
Invoice Generation and Management
- Automatic invoice generation for completed bookings.
- Storage and tracking of invoices for users.
Notification System
- Delivery of notifications for booking confirmations and updates.
- Personalization options for notification preferences.
Entities and Attributes for Booking and Reservation Systems
- UserID (Primary Key): Unique identifier for each user.
- Name: Name of the user.
- Email: Email of the user.
- Phone: Contact details of the user.
2. Services
- ServiceID (Primary Key): Unique identifier for each service.
- Name: Name of the service.
- Description: Description of the services.
- Price: Price of the service.

3. Bookings
- BookingID (Primary Key): Unique identifier for each booking.
- UserID (Foreign Key): UserID is a foreign key in a table that references the User table.
- ServiceID (Foreign Key): ServiceID is a foreign key in a table that references the Services table.
- Date: Date of the booking.
- Status: Status of booking whether it is confirmed, pending or cancelled.
4. Locations
- LocationID (Primary Key): Unique identifier for each location.
- Name: Name of the location.
- Address: Address of the location.
- City: City of the location.
- Country: Country of the location.
- ReviewID (Primary Key): Unique identifier for each review.
- ServiceID (Foreign Key): ServiceID is a foreign key in a table that references the Services Table.
- Rating: Rating which user gives.
- Comment: Comments given by users.
- PaymentID (Primary Key): Unique identifier for each payment.
- BookingID (Foreign Key): BookingID is a foreign key in a table that references the Booking table.
- Amount: Total amount of the payment.
- PaymentDate: Date of the payment.
- PaymentMethod: Type of the payment like UPI or credit card or cash.
7. Cancellation/Refund
- CancellationRefundID (Primary Key): Unique identifier for each cancellation or refund.
- CancellationDate: Date of cancellation.
- RefundAmount: Amount which will get refunded.
8. Invoices
- InvoiceID (Primary Key): Unique identifier for each Invoice.
- UserID (Foreign Key): UserID is a foreign key in a table that references the user table.
- Amount: Amount in the invoice.
- Date: Date of invoice.
9. Notifications
- NotificationID (Primary Key): Unique identifier for each notification.
- Message: Message in the notification.
Relationships between Entities
1. user – bookings relationship.
- One user can make multiple bookings (One-to-Many).
- Each booking is associated with one user.
- This relationship allows the system to track which user made a particular booking.
2. Services – Bookings Relationship
- One service can be booked multiple times (One-to-Many).
- Each booking is for one specific service.
- This relationship enables the system to link bookings to the services users have reserved.
3. Users – Reviews Relationship
- One user can write multiple reviews (One-to-Many).
- Each review is written by one user.
- This relationship connects users to the reviews they have submitted for services.
4. Services – Reviews Relationship
- One service can have multiple reviews (One-to-Many).
- Each review is associated with one specific service.
- This relationship allows users to leave feedback for the services they have utilized.
5. Services – Location Relationship
- Many services can be offered at one location (Many-to-One).
- Each service is located at one specific location.
- This relationship indicates where services are available, helping users find services based on their location preferences.
6. Bookings -Payment Relationship
- Each booking is associated with one payment (One-to-One).
- Each payment corresponds to one booking.
- This relationship allows the system to link bookings to the payments made by users, facilitating financial transactions tracking.
7. Bookings – Cancellation/Refund Relationship
- Each booking is associated with one cancellation or refund record (One-to-One).
- Each cancellation or refund record corresponds to one booking.
- This relationship enables the system to manage cancellation and refund processes for bookings, tracking relevant information such as cancellation dates and refund amounts.
8. User – Payment Relationship
- A user can make multiple payments.(One-to-Many)
- Each payment is made by one user.
9. Users – Invoices Relationship
- One user can have multiple invoices.(One-to-Many )
- This relationship signifies that a single user may have multiple invoices associated with their account.
10. Users – Notifications Relationship
- One user can receive multiple notifications.(One-to-Many)
- This relationship indicates that a user can receive multiple notifications from the system. Notifications may include booking confirmations, or updates on service availability.
ER Diagram of Booking and Reservation Systems
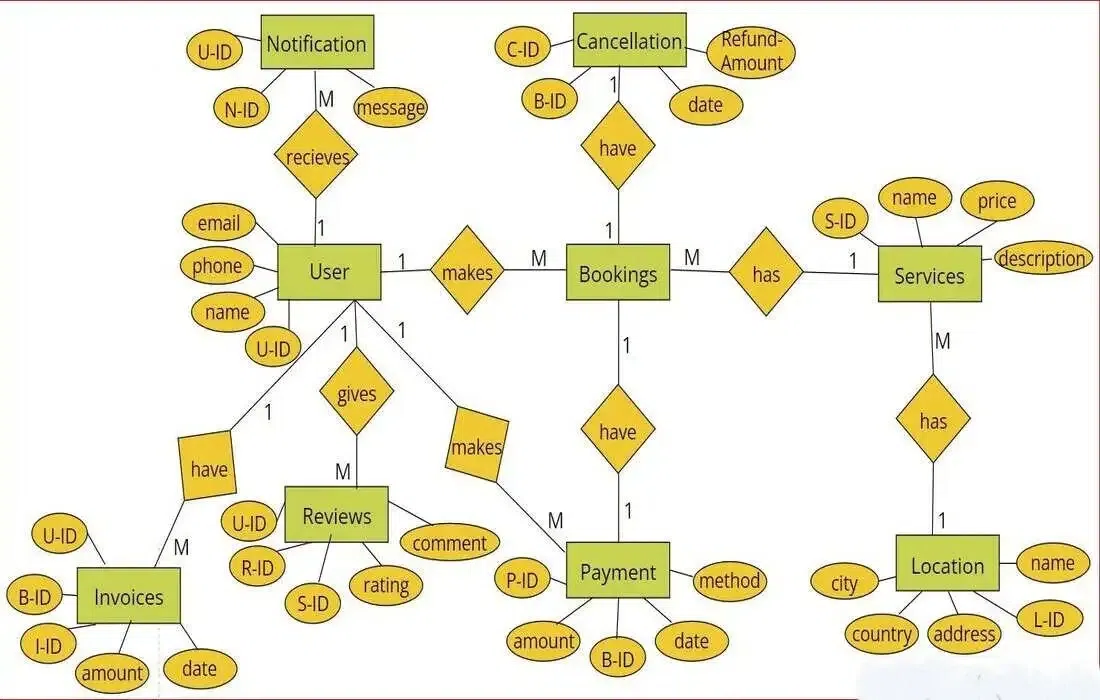
Entities in SQL Format
Tips & tricks to design a database.
- Normalize the database: Normalize the database to avoid the redundancy and the dependency.
- Use appropriate data types: Choose proper data types for attributes to ensure optimal storage and assure data integrity.
- Index key fields: Indexing primary and foreign key fields can provide better performance with queries.
- Implement constraints: Apply constraints like NOT NULL , UNIQUE , and FOREIGN KEY to ensure data integrity.
- Consider scalability: Design the database with scalability in mind so as to be able to accommodate future growth and adjust to changes in requirements.
- Optimize queries: Write effective SQL queries and factor them out for better performance.
- Document the design: Make sure to document database design in details for better understanding and maintenance in the future.
- Security measures: Implement security measures such as user authentication and authorization to prevent unauthorized access into sensitive data.
Introducing the ER Diagram of the booking and reservation systems requires paying attention to entities, attributes, relationships, normalization and concurrency control mechanisms. Well-developed ER diagrams provide a basis for making systems that are not only strong but also scalable, adequate for the changing purview of customers as well as the service providers. With the help of such diagrams, functionality and structure of the system is simplified, which increases the chances of successful implementation and smooth operation of booking and reservation systems no matter which industry they are used in.
Please Login to comment...
Similar reads.
- Dev Scripter
- Database Design
- Dev Scripter 2024
- Top 10 Fun ESL Games and Activities for Teaching Kids English Abroad in 2024
- Top Free Voice Changers for Multiplayer Games and Chat in 2024
- Best Monitors for MacBook Pro and MacBook Air in 2024
- 10 Best Laptop Brands in 2024
- 15 Most Important Aptitude Topics For Placements [2024]
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

Tourism Management System ER Diagram
Subscribe our youtube channel for latest project videos and tutorials click here.
- Posted By: freeproject
- Comments: 0
This ER (Entity Relationship) Diagram represents the model of Tourism Management System Entity. The entity-relationship diagram of Tourism Management System shows all the visual instrument of database tables and the relations between Travel Agent, Transportation, Customer, Hotel etc. It used structure data and to define the relationships between structured data groups of Tourism Management System functionalities. The main entities of the Tourism Management System are Customer, Travel Agent, Package, Transportation, Booking and Hotel.
Tourism Management System entities and their attributes :
- Customer Entity : Attributes of Customer are customer_id, customer_name, customer_mobile, customer_email, customer_username, customer_password, customer_address
- Travel Agent Entity : Attributes of Travel Agent are travel_agent_id, travel_agent_college_id, travel_agent_name, travel_agent_mobile, travel_agent_email, travel_agent_username, travel_agent_password, travel_agent_address
- Package Entity : Attributes of Package are package_id, package_tour_id, package_name, package_amount, package_total, package_type, package_description
- Transportation Entity : Attributes of Transportation are transportation_id, transportation_tour_id, transportation_name, vtype, vdescription
- Booking Entity : Attributes of Booking are booking_id, booking_hotel_id, booking_title, booking_type, booking_date, booking_description
- Hotel Entity : Attributes of Hotel are hotel_id, hotel_name, hotel_type, hotel_rent, hotel_description, hotel_address
Description of Tourism Management System Database :
- The details of Customer is store into the Customer tables respective with all tables
- Each entity ( Hotel, Package, Booking, Travel Agent, Customer) contains primary key and unique keys.
- The entity Package, Booking has binded with Customer, Travel Agent entities with foreign key
- There is one-to-one and one-to-many relationships available between Booking, Transportation, Hotel, Customer
- All the entities Customer, Booking, Package, Hotel are normalized and reduce duplicacy of records
- We have implemented indexing on each tables of Tourism Management System tables for fast query execution.
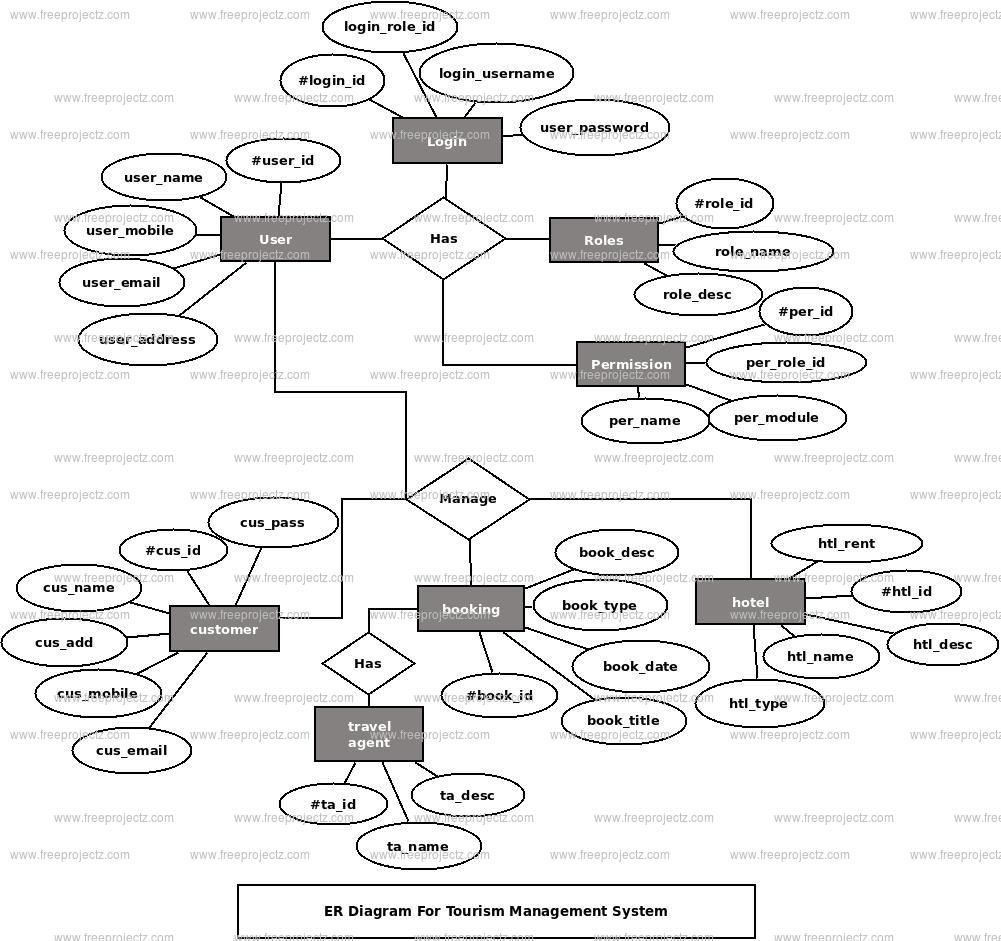
Tourism Management System ER Diagram Project Source Code and Database
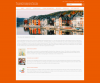
Related Tourism Management System ER Diagram Projects
Project category.
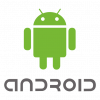
Related Entity Relationship Diagram (ERD)
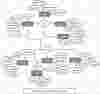
Railway Management System ER Diagram
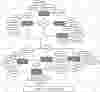
Crime Reporting System ER Diagram
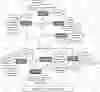
Farm Management System ER Diagram
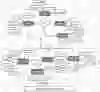
RTO Registration System ER Diagram
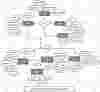
Movie Library Management System ER Diagram
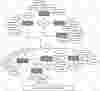
Garment Shop Automation ER Diagram
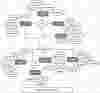
Ecare System ER Diagram
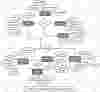
Appartment Maintance Management System ER Diagram
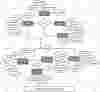
Canteen Billing System ER Diagram

Travel Agency ER Diagram.vpd
You may also like.
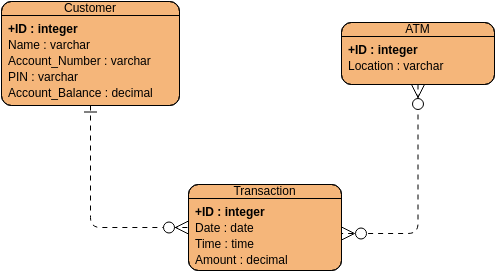
Create beautiful designs on-the-fly
No credit card required. No contracts to cancel. No downloads. No hidden costs.
©2024 by Visual Paradigm. All rights reserved.
- Terms of Service
- Privacy Policy
- Security Overview
You are using an outdated browser. Upgrade your browser today or install Google Chrome Frame to better experience this site.
Entity Relationship Diagram For Travel Agency
Powerful drawing solution.
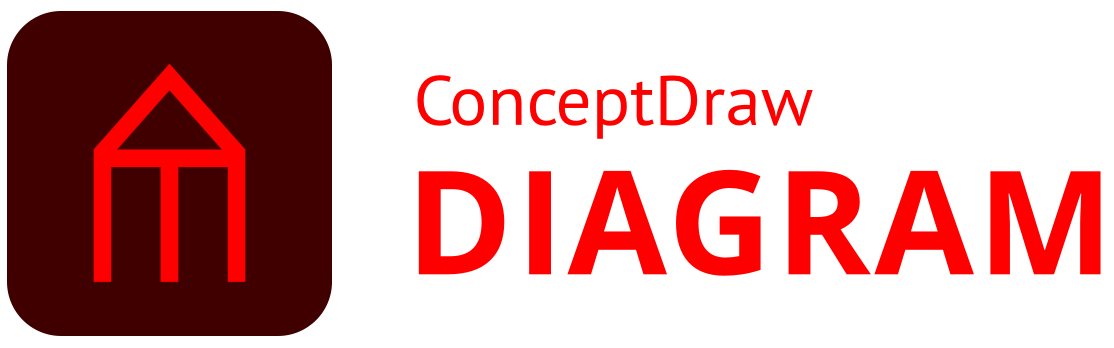
- Er Diagram For Travel Management System
- Er Diagram Tour And Travel Agency
- Er Diagram Of Tour And Travel Management System
- ER Diagram Styles | Components of ER Diagram | Top 5 Android ...
- Data Flow Diagram Of Sales For Travel Agency
- Er Diagram For Tour And Travel Management System
- Er Diagram For Tour Management
- Tours And Travels Management System In Dfd Diagram
- Entiy Relationship Diagram For Travel Mngmnt Systm
- Draw A Data Flow Diagram Level 1 Of Travel Agent Booking System
- ER Diagram For Class Management System
- Traveling Agency Dfd Diagram
- Cab booking public process - Collaboration BPMN 2.0 diagram ...
- UML Class Diagram Example - Social Networking Site | UML ...
- UML Class Diagram Example - Social Networking Site | UML Use ...
- Travel Management System Dfd Diagram
- Data Flow Diagram Of Travel Management System
- Business Process Modeling Software for Mac | BPMN 2.0 | Entity ...
- UML Collaboration Diagram (UML2.0) | Diagramming Software for ...
- BPMN 2.0 | BPMN | Business Process Modeling Software for Mac ...
- ERD | Entity Relationship Diagrams, ERD Software for Mac and Win
- Flowchart | Basic Flowchart Symbols and Meaning
- Flowchart | Flowchart Design - Symbols, Shapes, Stencils and Icons
- Flowchart | Flow Chart Symbols
- Electrical | Electrical Drawing - Wiring and Circuits Schematics
- Flowchart | Common Flowchart Symbols


COMMENTS
Learn how to design ER diagrams for a database that supports user registration, search, booking, and management of flights, accommodations, and activities. See the entities, attributes, and relationships involved in a Travel and Tourism Booking System and their ER diagram.
The entity-relationship diagram of Travel and Travel Management System shows all the visual instrument of database tables and the relations between Transportation, Hotel, Package, Customers etc. It used structure data and to define the relationships between structured data groups of Travel and Travel Management System functionalities.
About ER diagrams. We often make an entity-relationship (ER) diagram, ERD, or entity-relationship model, in the early stages of designing a database. An ERD is perfect for quickly sketching out the elements needed in the system. The ERD explains how the elements interact. ER diagrams can be shared with colleagues.
relational model.pdf. requirements document.PDF. tour-management-system. /. ER DIAGRAM.pdf. Cannot retrieve latest commit at this time. History. 335 KB. system that automates the process and activities of travel agencies and stores customer details. - tour-management-system/ER DIAGRAM.pdf at master · anan-123/tour-management-system.
Online Tourism Management System in PHP with source code is free to download, Use for educational purposes only! After Starting Apache and MySQL in XAMPP, follow the following steps. 1st Step: Extract file. 2nd Step: Copy the main project folder. 3rd Step: Paste in xampp/htdocs/.
The entity-relationship diagram of Travel Agency Management System shows all the visual instrument of database tables and the relations between Customer, Bookings, Travel Agency, Payments etc. It used structure data and to define the relationships between structured data groups of Travel Agency Management System functionalities. The main ...
1. ER DIAGRAM FOR A TRAVEL ITINERARY PLANNER. Entity: The entities are User, Itinerary, Destination, Transportation, Accommodation and Payment.. Attributes: The attributes are User ID, Name, Phone ...
The user needs to visit the travel agency office to plan any tour. It involves a lot of manual paperwork, and customers need to stay in the queue for a long time. ... Time-Saving: One can access the travel management office from anywhere on the web and need not visit the travel agency office. ... Flowchart of the system. ER diagram: Fig. 2: ER ...
ER Diagram of Tourism Management System. Nearly everyone goes on a vacation and a Tourism management system would play a vital role in planning the perfect trip. The tourism management system ...
This document provides details about a project for developing a Tour and Travel Management System. It includes information about the objectives, features, existing system, need for a new system, modules, hardware and software requirements, system flowchart, timeline, UML diagrams, data dictionary, input design, and testing plan. The main objective is to provide online registration, tour ...
This document provides an overview of the tour and travel management system project documentation. It discusses features such as centralized cryptography key repositories, validation processes, custom CSS, and technology used. The documentation describes aspects like data flow, authentication, password recovery, and various modules within the system for managing tours, travel packages ...
The entity-relationship diagram of Travel Management System shows all the visual instrument of database tables and the relations between Customer, Bookings, Travel Agency, Payments etc. It used structure data and to define the relationships between structured data groups of Travel Management System functionalities. The main entities of the ...
An Entity-Relationship Diagram (ERD) is a visual presentation of entities and relationships. That type of diagrams is often used in the semi-structured or unstructured data in databases and information systems. At first glance ERD is similar to a flowch Er Diagram For Tour Management
Use Creately's easy online diagram editor to edit this diagram, collaborate with others and export results to multiple image formats. You can easily edit this template using Creately. You can export it in multiple formats like JPEG, PNG and SVG and easily add it to Word documents, Powerpoint (PPT) presentations, Excel or any other documents.
Database Design for Booking and Reservation Systems. This platform includes a booking and reservation system that supports all aspects of handling bookings, and payments, as well as user management and interactions. Users are able to register and browse through the list of services. The system does all in all servicing related listings, as such ...
ABSTRACT This project < TOUR AND TRAVEL MANAGEMENT SYSTEM = is used to automate all process of the tour and travel, which deals with creation, booking and confirmation and user details. The project is designed HTML-PHP as front end and MySQL as backend which works in any browsers. ... Figure 27 ER Diagram; Table 1 Budget breakdown LIST OF ...
Tours & Travel Management System is an application will help in maintaining the operations . performed related to sight-seeing and travelling. Most of the people in this world like to travel ... ER Diagrams Implementation . 4.1.1 Data Flow Diagram . A Data Flow Diagram (DFD) is a diagram that describes the flow of data and the .
PHP Projects on Online Tourism Portal. Get 6 diagrams only in 300/- or $6.99 USD. Call or WhatsApp us on +91-8470010001 for more details. This ER (Entity Relationship) Diagram represents the model of Railway Management System Entity. The entity-relationship diagram of Railway Management System shows all the visual instrument of database tables ...
Administrator module is second important module of this project. Administrator provides booking (Hotel, Guide, Transportation) informations to the users. OBJECTIVE: The objective of the project is to develop a system that automates the processes and activities of a travel and tourism agency.
Travel Agency ER Diagram.vpd. Designed by @Dhaivat3212 Edit this Design.
Er Diagram Tour And Travel Agency. Bpmn Diagram Of Travel Agency System. Er Diagram For Tour And Travel Management System. Dfd For Travel Agency Management System. Tours And Travels Management System In Dfd Diagram. Uml Diagrams For Tours And Travels Management System. Er Diagram For Tour Management. ER Diagram For Class Management System.
Er Diagram For Tour Management. Tours And Travels Management System In Dfd Diagram. Entiy Relationship Diagram For Travel Mngmnt Systm. Draw A Data Flow Diagram Level 1 Of Travel Agent Booking System. ER Diagram For Class Management System. Traveling Agency Dfd Diagram.